Let’s Break Down the “Star Flight” Scene
Of all the puzzle scenes we have in our Modulo Code “Code E.D.” lesson, the “Star Flight” scene surprises many of our users the most. We think it’s possibly due to a combination of a few things that makes this scene looks curiously harder than what it actually is.
It might have been that this scene comes relatively early in the lessons soon after we introduce the concept of loops, or that the stage in the scene just looks overwhelmingly tedious to some, with too many markers to visit. The scene mission statement that starts with “This puzzle has nested patterns…” probably makes it sound even harder.
Let’s start with an understanding that this puzzle does follow a certain pattern and that the pattern is repetitive. The scene’s mission text gives a big clue as to how to break down its complexity when it says “By repeating the solution that solves each quadrant of the stage four times, the whole puzzle can be solved…”. We can imagine that perhaps each quadrant of the stage is a pattern that, once solved, we could probably reuse the solution to solve other quadrants and then the whole puzzle.
With that intuition, let’s look at the quadrant in front of the robot to its left. Now, look even closer to just that one section with the marker nearest to the robot and ask yourself this question: “How do I move the robot so that it passes the nearest marker then returns to its starting location?”. The code that does that would look like the following:
ed:moveForward() ed:turnLeft() ed:jump() ed:turnLeft() ed:jump() ed:turnLeft() ed:moveForward() ed:turnLeft()
When you run this code, the robot travels one rotation counter-clockwise before returning to the same starting location at the center of the stage. It rolls forward and jumps along its traveling path.
All is good! Let’s now understand the code we just wrote and try to see how we can extend it to reach the markers on the second and third stair level of the stage, still within the same quadrant. For example, to move the robot past the marker on the second stair level, we would extend the code to something like this:
ed:moveForward() ed:moveForward() ed:turnLeft() ed:jump() ed:jump() ed:turnLeft() ed:jump() ed:jump() ed:turnLeft() ed:moveForward() ed:moveForward() ed:turnLeft()
Did you notice a pattern here? That’s right. It’s still the same code but with the move and jump actions repeated. To move the robot past the marker on the third stair level, we would repeat the robot movement actions three times. Knowing that we’ll need to make three rotations, each rotation for each stair level, we can now write a more generic version of this code using loops. This code moves the robot past all the markers in this quadrant and brings it back to the center of the stage.
for rotation = 1,3,1 do for step = 1,rotation,1 do ed:moveForward() end ed:turnLeft() for step = 1,rotation,1 do ed:jump() end ed:turnLeft() for step = 1,rotation,1 do ed:jump() end ed:turnLeft() for step = 1,rotation,1 do ed:moveForward() end ed:turnLeft() end
Notice that we’ve just solved part of the puzzle for the quadrant in front of the robot to its left. An important insight here is that if only we can re-orient the robot in a different direction, we could use the same code to solve another quadrant the same way we solve the first one! This insight allows us to not only solve for the second quadrant, but for all four of them with a simple loop that repeats four times, each iteration for each quadrant of the stage. Here we have our final solution. Voila! Feel free to debug the following code line by line to understand it better.
for quad = 1,4,1 do for rotation = 1,3,1 do for step = 1,rotation,1 do ed:moveForward() end ed:turnLeft() for step = 1,rotation,1 do ed:jump() end ed:turnLeft() for step = 1,rotation,1 do ed:jump() end ed:turnLeft() for step = 1,rotation,1 do ed:moveForward() end ed:turnLeft() end ed:turnLeft() end
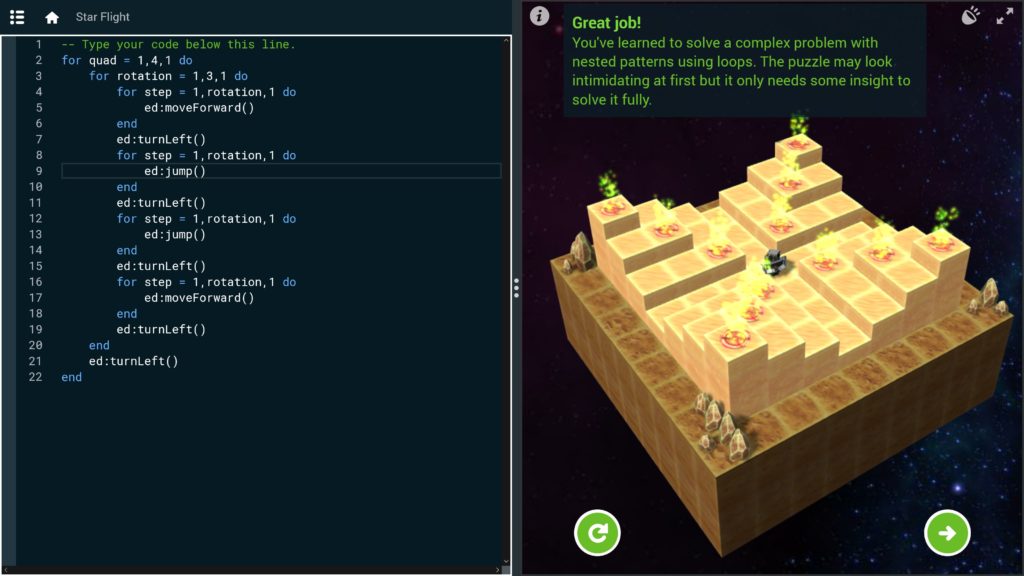